How Builders Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
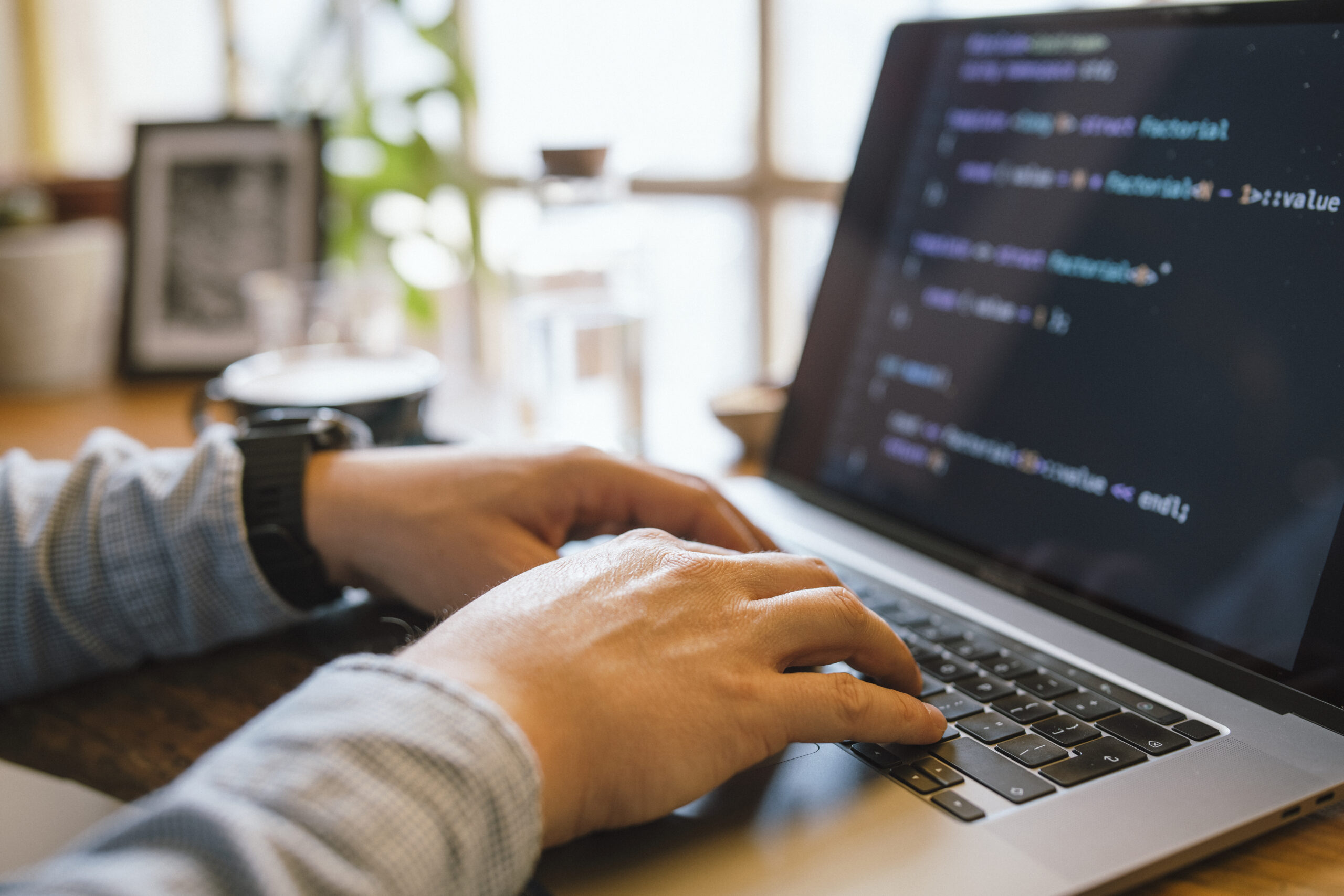
Debugging is one of the most vital — nonetheless often disregarded — capabilities in a very developer’s toolkit. It isn't really pretty much repairing broken code; it’s about knowledge how and why points go Completely wrong, and learning to think methodically to solve problems efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures that will help builders degree up their debugging sport by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies developers can elevate their debugging expertise is by mastering the tools they use everyday. When composing code is one particular A part of development, recognizing tips on how to communicate with it successfully all through execution is Similarly essential. Modern progress environments arrive equipped with highly effective debugging capabilities — but lots of developers only scratch the surface area of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code about the fly. When utilized effectively, they Allow you to notice precisely how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-conclusion developers. They assist you to inspect the DOM, check community requests, view real-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform irritating UI difficulties into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging functionality issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation control methods like Git to grasp code heritage, obtain the precise moment bugs had been introduced, and isolate problematic adjustments.
Eventually, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings in order that when troubles occur, you’re not dropped in the dead of night. The greater you already know your applications, the greater time you may shell out resolving the particular dilemma as an alternative to fumbling by means of the method.
Reproduce the trouble
Probably the most crucial — and often overlooked — steps in helpful debugging is reproducing the condition. Right before jumping into the code or making guesses, builders need to have to make a steady atmosphere or scenario wherever the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a problem is accumulating as much context as feasible. Question queries like: What steps brought about the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the exact ailments below which the bug takes place.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting a similar info, simulating identical user interactions, or mimicking technique states. If the issue appears intermittently, look at creating automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The difficulty may be surroundings-precise — it'd occur only on certain working programs, browsers, or underneath particular configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It needs persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible situation, You should utilize your debugging applications extra correctly, exam potential fixes safely, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance into a concrete problem — and that’s where builders thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, builders should really study to take care of error messages as direct communications from the procedure. They generally inform you precisely what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Quite a few developers, specially when underneath time stress, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root result in. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging approach.
Some errors are obscure or generic, As well as in Those people instances, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and recent variations in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most impressive applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what level. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard activities (like effective start-ups), Alert for likely concerns that don’t break the application, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on vital functions, state variations, enter/output values, and critical final decision factors in your code.
Structure your log messages clearly and continually. Contain context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your purposes, and Enhance the In general maintainability and reliability of the code.
Assume Like a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and take care of bugs, developers should technique the method similar to a detective resolving a secret. This mindset aids break down intricate difficulties into workable pieces and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Think about the indications of the problem: error messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, form hypotheses. Ask your self: What might be creating this behavior? Have any changes a short while ago been built into the codebase? Has this challenge transpired ahead of beneath equivalent situations? The goal should be to slender down opportunities and discover prospective culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled environment. For those who suspect a certain operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and Enable the outcome lead you nearer to the truth.
Pay back near notice to compact information. Bugs frequently disguise while in the least predicted locations—similar to a missing semicolon, an off-by-one mistake, or a race affliction. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may possibly hide the true challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering hidden concerns in advanced units.
Create Exams
Composing assessments is among the simplest ways to boost your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early but in addition function a security Internet that provides you self esteem when earning variations to your codebase. A well-tested application is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with multiple parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Crafting assessments also forces you to Consider critically regarding your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and check out your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you catch additional bugs, faster and even more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the issue—looking at your display screen for hrs, hoping Alternative following Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code which you wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
If you’re caught, a great guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath limited deadlines, nonetheless it basically contributes to a lot quicker and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a sensible strategy. It provides your Mind space to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key concerns once the bug is resolved: What triggered it? Why did it go unnoticed? Could it have already been caught previously with greater procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be especially impressive. No matter if it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals read more steer clear of the very same concern boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. In any case, some of the ideal developers will not be those who compose fantastic code, but individuals who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become superior at Anything you do.